Design Pattern
Provide/Inject
When managing data between parent and child components, Vue gives us the ability to use something known as props to pass data down from parent to child. Props can only flow in one direction, from parent components to child components (and further down). When state changes occur on parent elements, Vue will re-render components that depend on those values.
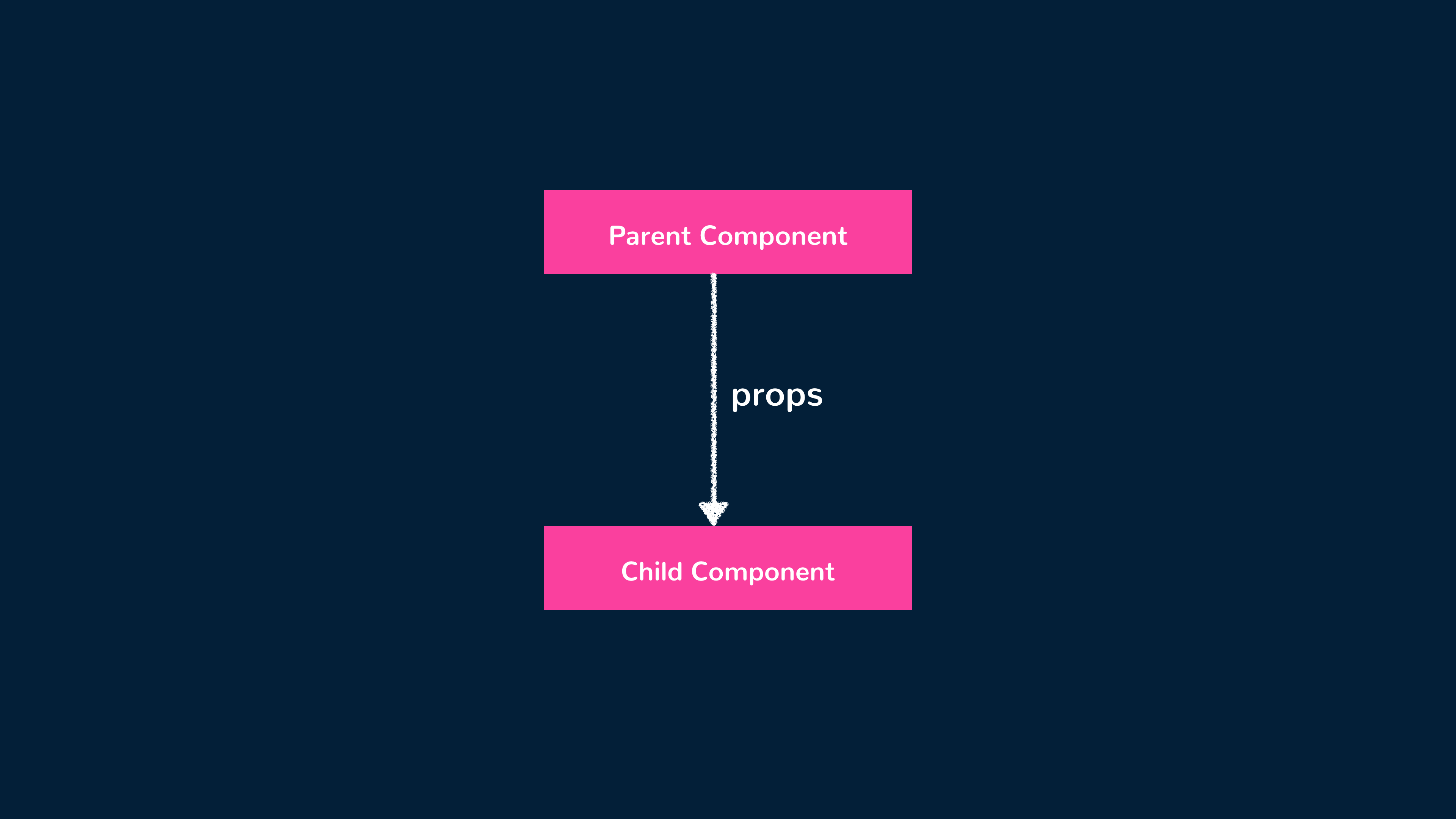
Using props works well in most cases. However, when working in large applications with a large number of components in the component tree, props can become hard to maintain since props need to be declared in each and every component in the component tree.
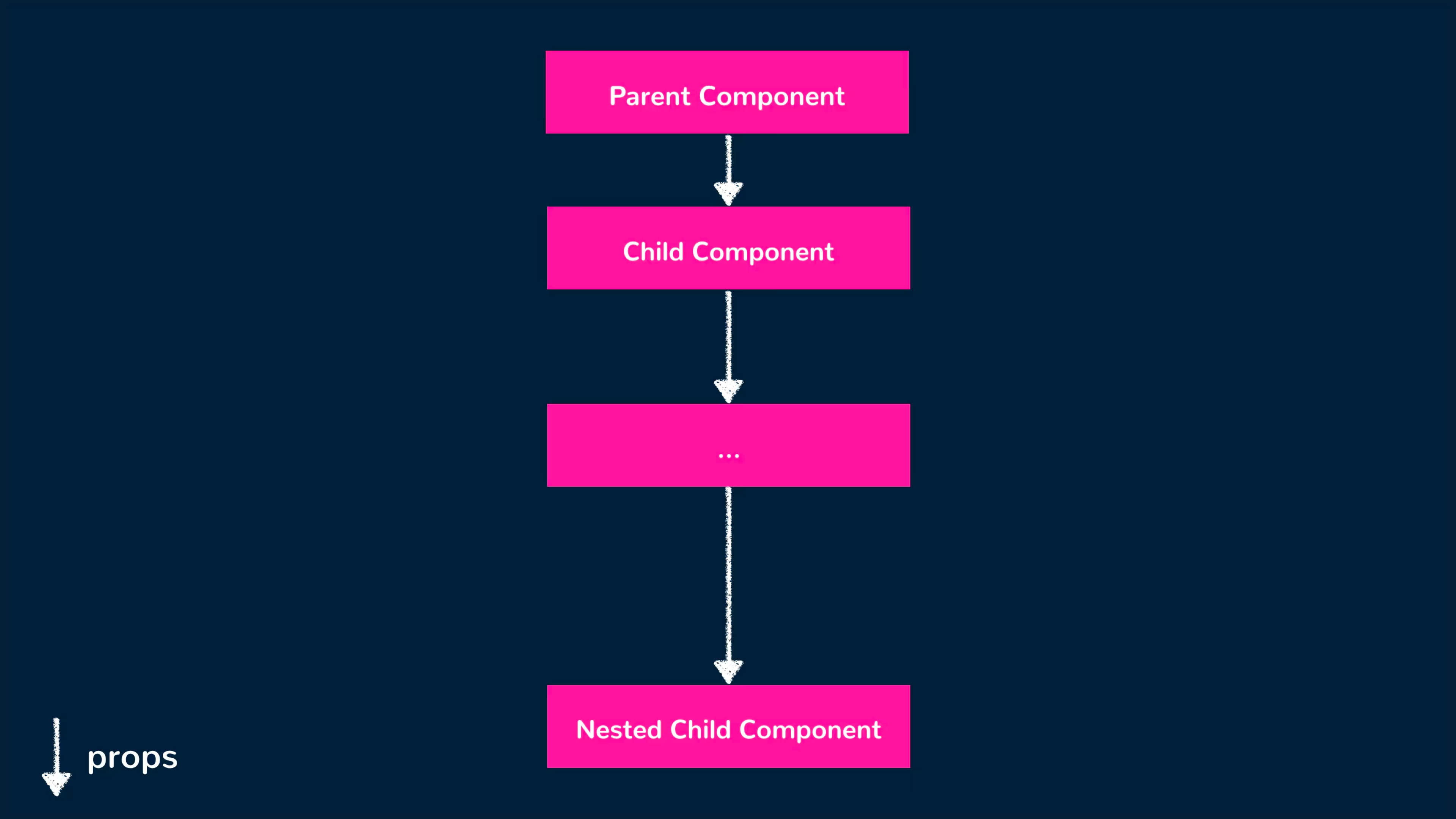
When considering how data can be managed between a large number of components, it’s often best to work towards a solution that allows the management of application-level state in a maintainable and manageable manner (e.g. creating a reusable store, using Pinia, etc.). We talk about this in more detail in the State Management guide.
However, Vue also provides a certain pattern to help avoid the need for complex prop drilling in a Vue application known as the provide/inject pattern.
Provide/Inject
The provide()
function in Vue allows us to pass data through a component tree without the need to prop-drill (i.e., pass props down
manually at every level). On the other hand, the inject()
option is used in child components to access the provided data or methods from
their parent or any ancestor component.
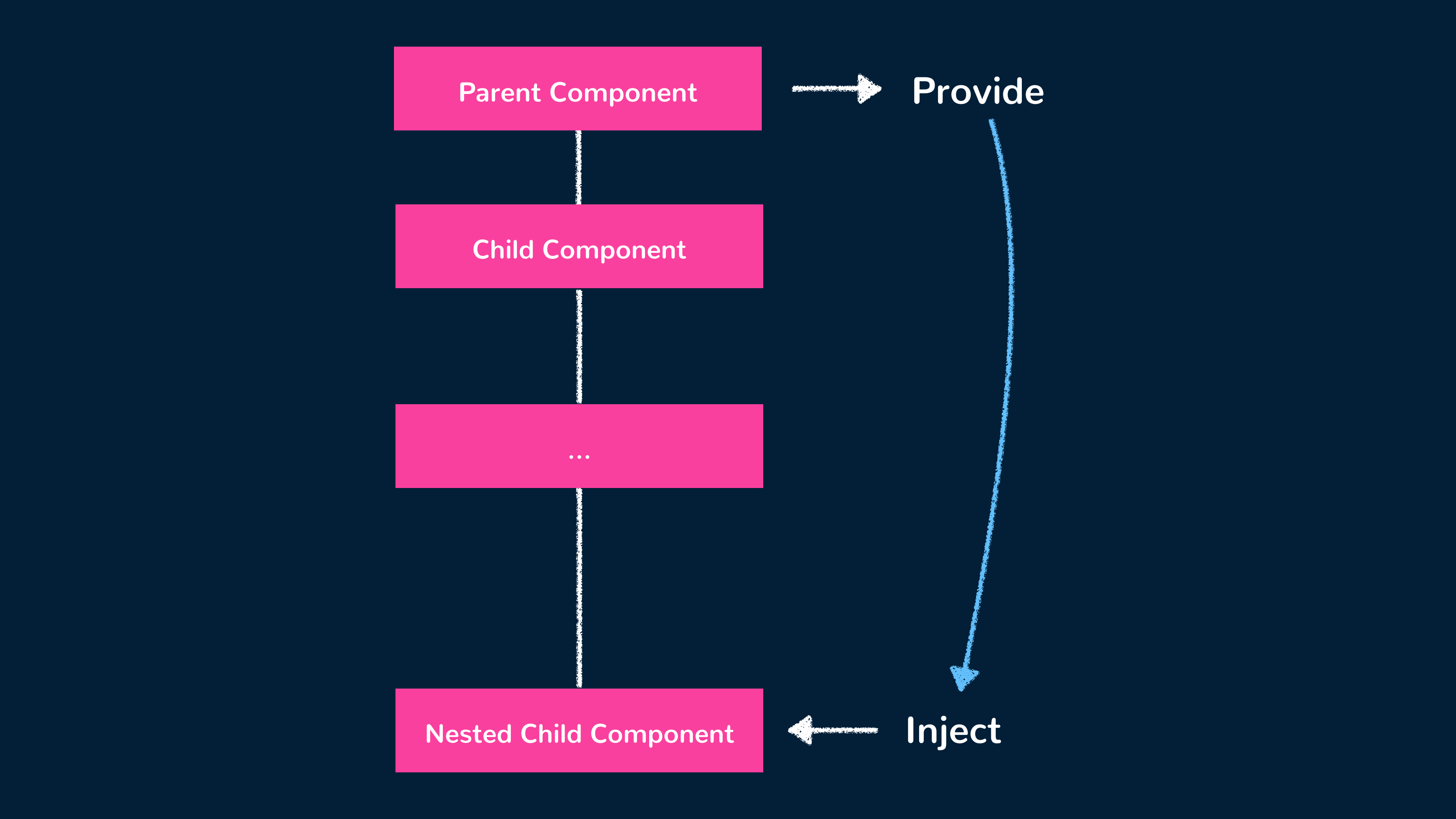
We’ll go through a simple example to illustrate how this can be done. Suppose we have a parent component called App
that wants to share a
piece of data with its child component, ChildComponent
. Instead of passing this data as a prop, we can use provide()
in the parent component
to make the data available to all its child components.
<template>
<div id="app">
<ChildComponent />
</div>
</template>
<script setup>
import { provide } from "vue";
import ChildComponent from "./components/ChildComponent";
provide("data", "Data from parent!");
</script>
We can then access this provided data in the ChildComponent
with the help of the inject()
function.
<template>
<div>
<p>{{ data }}</p>
</div>
</template>
<script setup>
import { inject } from "vue";
const data = inject("data");
</script>
By specifying inject("data")
in the child component (ChildComponent
), we directly access the provided data
value from the parent component.
We then bind data
to the template to display its value.
1<template>2 <div id="app">3 <ChildComponent />4 </div>5</template>67<script setup>8import { provide } from "vue";9import ChildComponent from "./components/ChildComponent";1011provide("data", "Data from parent!");12</script>
With provide/inject, we would notice the same behavior as we’ve seen above even if we had numerous child components within the component
hierarchy tree. As an example, assume we had <ChildComponent />
, <ChildComponent2 />
, <ChildComponent3 />
, <ChildComponent4 />
and
<ChildComponent5 />
components where each child component is a parent to the other.
<!-- ChildComponent5 -->
<template>
<div>
<p>{{ data }}</p>
</div>
</template>
<script setup>
import { inject } from "vue";
const data = inject("data");
</script>
<!-- ------------- -->
<!-- ChildComponent4 -->
<template>
<ChildComponent5 />
</template>
<script setup>
import ChildComponent5 from "./ChildComponent5";
</script>
<!-- ------------- -->
<!-- ChildComponent3 -->
<template>
<ChildComponent4 />
</template>
<script setup>
import ChildComponent4 from "./ChildComponent4";
</script>
<!-- ------------- -->
<!-- ChildComponent2 -->
<template>
<ChildComponent3 />
</template>
<script setup>
import ChildComponent3 from "./ChildComponent3";
</script>
<!-- ------------- -->
<!-- ChildComponent -->
<template>
<ChildComponent2 />
</template>
<script setup>
import ChildComponent2 from "./ChildComponent2";
</script>
<!-- ------------- -->
<!-- App -->
<template>
<div id="app">
<ChildComponent />
</div>
</template>
<script setup>
import { provide } from "vue";
import ChildComponent from "./components/ChildComponent";
provide("data", "Data from parent!");
</script>
<!-- ------------- -->
Data from the parent <App />
component will be rendered in the <ChildComponent5 />
component without the need to prop-drill data through
every component in the tree, thanks to provide/inject!
1<template>2 <div id="app">3 <ChildComponent />4 </div>5</template>67<script setup>8import { provide } from "vue";9import ChildComponent from "./components/ChildComponent";1011provide("data", "Data from parent!");12</script>
In addition to being able to provide()
data from a parent component, we can lift the provide()
up to the app level as well (i.e. where we
instantiate our Vue application).
import { createApp } from "vue";
import App from "./App.vue";
import "./styles.css";
const app = createApp(App);
// app-level provide
app.provide("data", "Data from parent!");
app.mount("#app");
Since app-level provides make data available to all components, they are often helpful when creating plugins — self-contained code that adds functionality to the entire Vue app.
Props vs. provide/inject
When do we choose between props and the provide/inject pattern? Both approaches have their advantages and disadvantages.
With props:
- We follow a clear pattern of passing data incrementally from one level to another (advantage).
- However, if our component hierarchy tree contains a large number of components, the process of passing props data one level at a time can become cumbersome (disadvantage).
With provide/inject
- Child components can directly access data from parent components located multiple levels above, eliminating the need for passing down data at each level (advantage).
- However, when bugs arise, debugging can be more challenging with provide/inject. This challenge becomes more pronounced in large-scale applications with numerous different providers (disadvantage).
The provide/inject pattern is most suitable for application-wide client data, such as theme information, locale/language preferences, and user authentication details. These types of data are better managed with provide/inject since any component within the application may require access to them at any given time.
On the other hand, props are ideal when data needs to be isolated within a specific set of components only.