Rendering Pattern
Streaming Server-Side Rendering
We can reduce the Time To Interactive while still server rendering our application by streaming server rendering the contents of our application. Instead of generating one large HTML file containing the necessary markup for the current navigation, we can split it up into smaller chunks! Node streams allow us to stream data into the response object, which means that we can continuously send data down to the client. The moment the client receives the chunks of data, it can start rendering the contents.
React’s built-in renderToNodeStream
makes it possible for us to send our application in smaller chunks. As the client can start painting the UI when it’s still receiving data, we can create a very performant first-load experience. Calling the hydrate
method on the received DOM nodes will attach the corresponding event handlers, which makes the UI interactive!
Let’s say we have an app that shows the user thousands of cat facts in the App
component!
1import React from "react";2import path from "path";3import express from "express";4import { renderToNodeStream } from "react-dom/server";56import App from "./src/App";78const app = express();910// app.get("/favicon.ico", (req, res) => res.end());11app.use("/client.js", (req, res) => res.redirect("/build/client.js"));1213const DELAY = 500;14app.use((req, res, next) => {15 setTimeout(() => {16 next();17 }, DELAY);18});1920const BEFORE = `21<!DOCTYPE html>22 <html>23 <head>24 <title>Cat Facts</title>25 <link rel="stylesheet" href="/style.css">26 <script type="module" defer src="/build/client.js"></script>27 </head>28 <body>29 <h1>Stream Rendered Cat Facts!</h1>30 <div id="approot">31`.replace(/32s*/g, "");3334app.get("/", async (request, response) => {35 try {36 const stream = renderToNodeStream(<App />);37 const start = Date.now();3839 stream.on("data", function handleData() {40 console.log("Render Start: ", Date.now() - start);41 stream.off("data", handleData);42 response.useChunkedEncodingByDefault = true;43 response.writeHead(200, {44 "content-type": "text/html",45 "content-transfer-encoding": "chunked",46 "x-content-type-options": "nosniff"47 });48 response.write(BEFORE);49 response.flushHeaders();50 });51 await new Promise((resolve, reject) => {52 stream.on("error", err => {53 stream.unpipe(response);54 reject(err);55 });56 stream.on("end", () => {57 console.log("Render End: ", Date.now() - start);58 response.write("</div></body></html>");59 response.end();60 resolve();61 });62 stream.pipe(63 response,64 { end: false }65 );66 });67 } catch (err) {68 response.writeHead(500, {69 "content-type": "text/pain"70 });71 response.end(String((err && err.stack) || err));72 return;73 }74});7576app.use(express.static(path.resolve(__dirname, "src")));77app.use("/build", express.static(path.resolve(__dirname, "build")));7879const listener = app.listen(process.env.PORT || 2048, () => {80 console.log("Your app is listening on port " + listener.address().port);81});
The App
component gets stream rendered using the built-in renderToNodeStream
method. The initial HTML gets sent to the response object alongside the chunks of data from the App component,
1<!DOCTYPE html>2<html>3 <head>4 <title>Cat Facts</title>5 <link rel="stylesheet" href="/style.css" />6 <script type="module" defer src="/build/client.js"></script>7 </head>8 <body>9 <h1>Stream Rendered Cat Facts!</h1>10 <div id="approot"></div>11 </body>12</html>
This data contains useful information that our app has to use in order to render the contents correctly, such as the title of the document and a stylesheet. If we were to server render the App
component using the renderToString
method, we would have had to wait until the application has received all data before it can start loading and processing this metadata. To speed this up, renderToNodeStream
makes it possible for the app to start loading and processing this information as it’s still receiving the chunks of data from the App component!
To see more examples on how to implement Progressive Hydration and Server Rendering, visit this GitHub repo.
See how styled-components use streaming rendering to optimize the delivery of stylesheets
Concepts
Like progressive hydration, streaming is another rendering mechanism that can be used to improve SSR performance. As the name suggests, streaming implies chunks of HTML are streamed from the node server to the client as they are generated. As the client starts receiving “bytes” of HTML earlier even for large pages, the TTFB is reduced and relatively constant. All major browsers start parsing and rendering streamed content or the partial response earlier. As the rendering is progressive, it results in a fast FP and FCP.
Streaming responds well to network backpressure. If the network is clogged and not able to transfer any more bytes, the renderer gets a signal and stops streaming till the network is cleared up. Thus, the server uses less memory and is more responsive to I/O conditions. This enables your Node.js server to render multiple requests at the same time and prevents heavier requests from blocking lighter requests for a long time. As a result, the site stays responsive even in challenging conditions.
React for Streaming
React introduced support for streaming in React 16 released in 2016. The following API’s were included in the ReactDOMServer to support streaming.
-
ReactDOMServer.renderToNodeStream(element)
: The output HTML from this function is the same asReactDOMServer.renderToString(element)
but is in a Node.js readablestream format instead of a string. The function will only work on the server to render HTML as a stream. The client receiving this stream can subsequently call ReactDOM.hydrate() to hydrate the page and make it interactive. -
ReactDOMServer.renderToStaticNodeStream(element)
: This corresponds toReactDOMServer.renderToStaticMarkup(element)
. The HTML output is the same but in a stream format. It can be used for rendering static, non-interactive pages on the server and then streaming them to the client.
The readable stream output by both functions can emit bytes once you start reading from it. This can be achieved by piping the readable stream to a writable stream such as the response object. The response object progressively sends chunks of data to the client while waiting for new chunks to be rendered.
Putting it all together, let us now look at the code skeleton for this as published here.
1import { renderToNodeStream } from 'react-dom/server';2import Frontend from '../client';34app.use('*', (request, response) => {5 // Send the start of your HTML to the browser6 response.write('<html><head><title>Page</title></head><body><div id="root">');78 // Render your frontend to a stream and pipe it to the response9 const stream = renderToNodeStream(<Frontend />);10 stream.pipe(response, { end: 'false' });11 // Tell the stream not to automatically end the response when the renderer finishes.1213 // When React finishes rendering send the rest of your HTML to the browser14 stream.on('end', () => {15 response.end('</div></body></html>');16 });17});
A comparison between TTFB and First Meaningful Paint for normal SSR Vs Streaming is available in the following image.
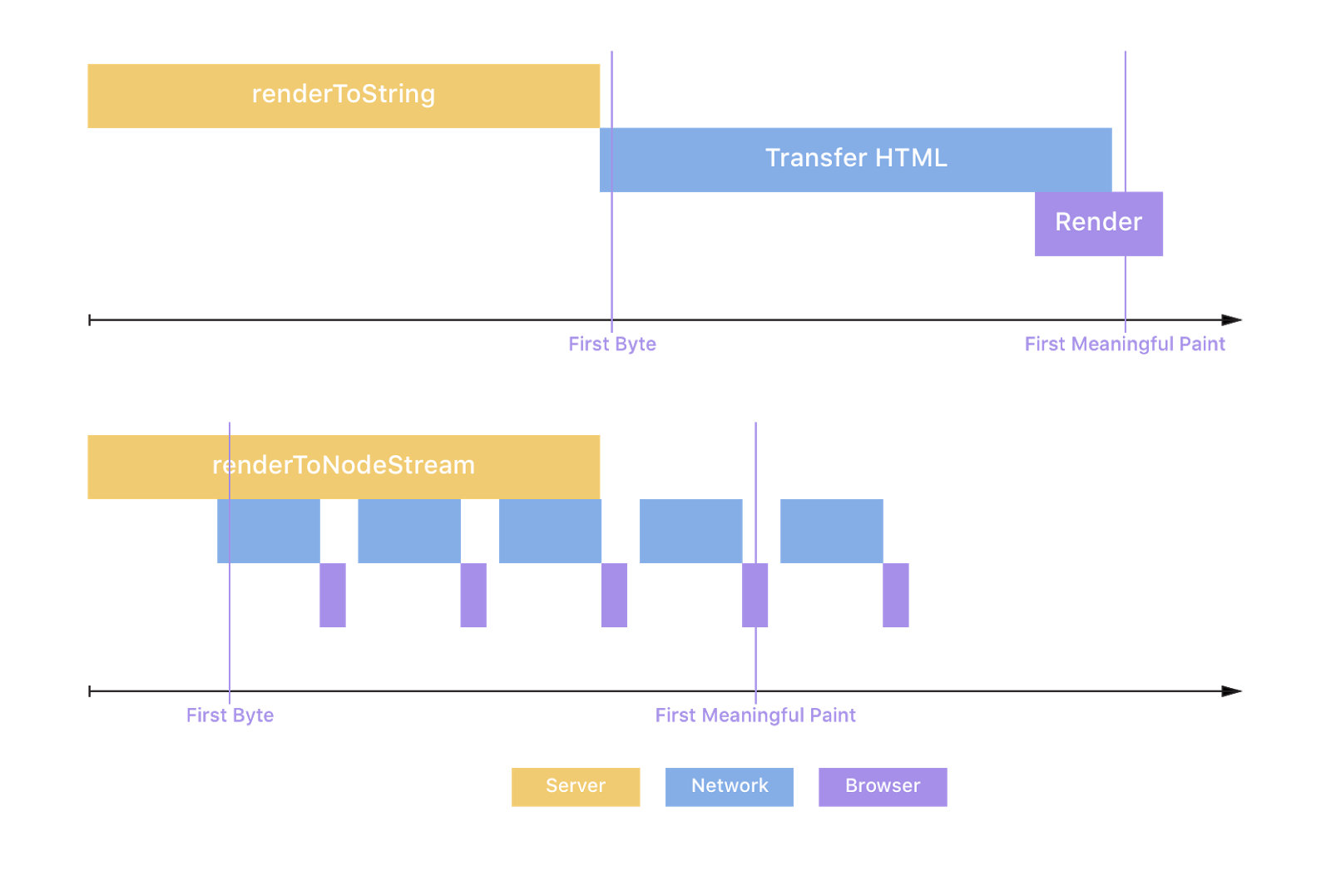
Image Source: https://mxstbr.com/thoughts/streaming-ssr/
Streaming SSR - Pros and Cons
Streaming aims to improve the speed of SSR with React and provides the following benefits
-
Performance Improvement: As the first byte reaches the client soon after rendering starts on the server, the TTFB is better than that for SSR. it is also more consistent irrespective of the page size. Since the client can start parsing HTML as soon as it receives it, the FP and FCP are also lower.
-
Handling of Backpressure: Streaming responds well to network backpressure or congestion and can result in responsive websites even under challenging conditions.
-
Supports SEO: The streamed response can be read by search engine crawlers, thus allowing for SEO on the website.
It is important to note that streaming implementation is not a simple
find-replace from renderToString
to renderToNodeStream()
. There are cases
where the code that works with SSR may not work as-is with streaming. Following
are some examples where migration may not be easy.
- Frameworks that use the server-render-pass to generate markup that needs
to be added to the document before the SSR-ed chunk. Examples are frameworks
that dynamically determine which CSS to add to the page in a preceding
<style>
tag, or frameworks that add elements to the document<head>
while rendering. A workaround for this has been discussed here. - Code, where renderToStaticMarkup is used to generate the page template and renderToString calls are embedded to generate dynamic content. Since the string corresponding to the component is expected in these cases, it cannot be replaced by a stream. An example of such code provided here is as follows.
res.write("<!DOCTYPE html>");
res.write(renderToStaticMarkup(
<html>
<head>
<title>My Page</title>
</head>
<body>
<div id="content">
{ renderToString(<MyPage/>) }
</div>
</body>
</html>);
Both Streaming and Progressive Hydration can help to bridge the gap between a pure SSR and a CSR experience. Let us now compare all the patterns that we have explored and try to understand their suitability for different situations.